- Home
- .NET coding challenges
- Using the yield statement in C#
Using the yield statement in C#
Yield in C# is an underused statement which can be used in an iterator to provide the next value or to signal the end of an iteration.
It can be used on collection types like a list or an array.
Microsoft provide a useful guide on how the yield statement works.
Take this code:
var oddNumbers = GetOddNumbers(new int[] { 1, 2, 3, 4, 5 });
foreach (var oddNumber in oddNumbers)
{
Console.WriteLine(oddNumber);
}
IEnumerable<int> GetOddNumbers(int[] numbers)
{
var oddNumbers = new List<int>();
foreach (int number in numbers)
{
if (number %2 == 1)
{
oddNumbers.Add(number);
}
}
return oddNumbers;
}
The output of this in a Console application would be:
1
3
5
How could we replace the functionality in the GetOddNumbers
method to include the yield
statement?
Give us your anonymous feedback regarding this page, or any other areas of the website.
Related challenges
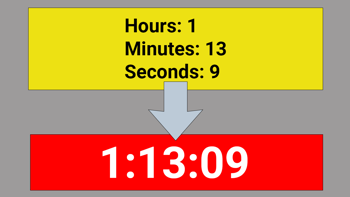
Convert hours, minutes and seconds into a time formatted string
Pass in the hours, minutes and seconds into a method and get it to return a time formatted string which should include leading zeros.
Contains online code editor
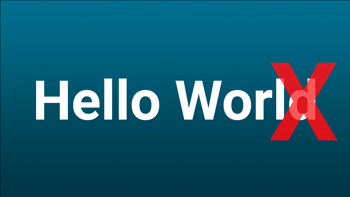
Removing the last character from a string
This coding challenge involves writing a C# method that will removing the last character from a string and returning the value.
Contains online code editor