- Home
- .NET coding challenges
- Convert hours, minutes and seconds into a time formatted string
Convert hours, minutes and seconds into a time formatted string
This coding challenge will see you pass in the hours, minutes and seconds into a method and convert it to a time formatted string.
Start off with this method:
public class TimeFunctions {
public string ConvertToTimeString(int hours, int minutes, int seconds)
{
if (
hours < 0 ||
minutes < 0 ||
minutes > 59 ||
seconds < 0 ||
seconds > 59
)
{
throw new ArgumentOutOfRangeException("Invalid parameters");
}
}
}
Go ahead and return the hours, minutes and seconds into a time formatted H:mm:ss
string.
For example, if you pass in hours = 1
, minutes = 32
and seconds = 33
, the method should return 1:32:33
.
We are already throwing an exception if any of the parameters are out of range.
Important things to consider:
- Include leading zeros on the minutes and seconds (but not the hours). So if you pass in
hours = 1
,minutes = 3
andseconds = 8
, the function should return1:03:08
. - If the
hours
you pass in is0
, it should still return the number of hours as part of the string. So if you pass inhours = 0
,minutes = 34
andseconds = 1
, the function should return0:34:01
.
Give us your anonymous feedback regarding this page, or any other areas of the website.
Related challenges
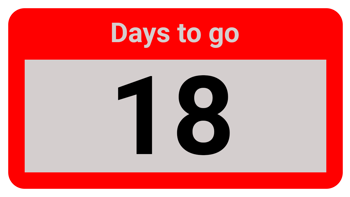
Work out the number of days between two dates
Write a C# function to work out the difference between two dates and return the number of days in this coding challenge.
Contains online code editor
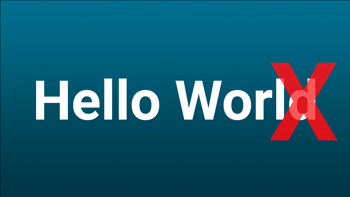
Removing the last character from a string
This coding challenge involves writing a C# method that will removing the last character from a string and returning the value.
Contains online code editor