- Home
- .NET coding challenges
- Using the abstract modifier in C#
How the abstract modifier works in a C# class
When using the abstract
modifier in C#, it indicates that the modifier has an incomplete implementation.
As well as a class, the abstract
modifier can be used in properties and methods.
A class with the abstract
modifier cannot be initalised directly. Instead, it needs to be inherited by a non-abstract class for it to be initalised.
Take this code:
public abstract class Currency
{
public abstract string Symbol { get; }
public abstract decimal USDConversationRate { get; }
}
public class USDollarsCurrency : Currency {
}
Why wouldn't this code snippet compile and what would we need to do to fix it?
Give us your anonymous feedback regarding this page, or any other areas of the website.
Related challenges
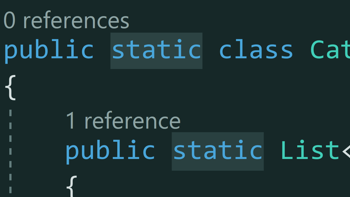
Add an extension method to a C# class
See if you can add a static extension method to a class and not modify the original type with our C# coding challenge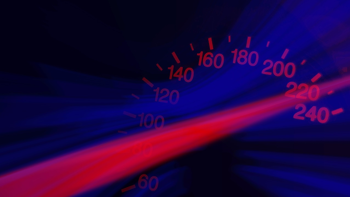
Write a C# function to convert MPH to KPH
Take our coding challenge to write a C# function with the necessary parameters that converts miles-per-hour (MPH) to kilometres-per-hour (KPH) and vice-versa.
Contains online code editor