Swagger dropped from .NET 9: What are the alternatives?
Published: Monday 9 December 2024
Microsoft have dropped support for Swagger UI package Swashbuckle in .NET 9.
They claim that the project is "no longer actively maintained by its community owner" and "issues have not been address or resolved".
This means that when you use the create a Web API using the .NET 9 template, you'll no longer have a UI to test out your API endpoints.
We'll investigate to see if we can use the Swagger UI in .NET 9 and whether there is a better alternative.
Creating a .NET project
Regardless of whether you create a .NET 8 or .NET 9 Web API using Visual Studio, you'll have the option to Enable OpenAPI support.
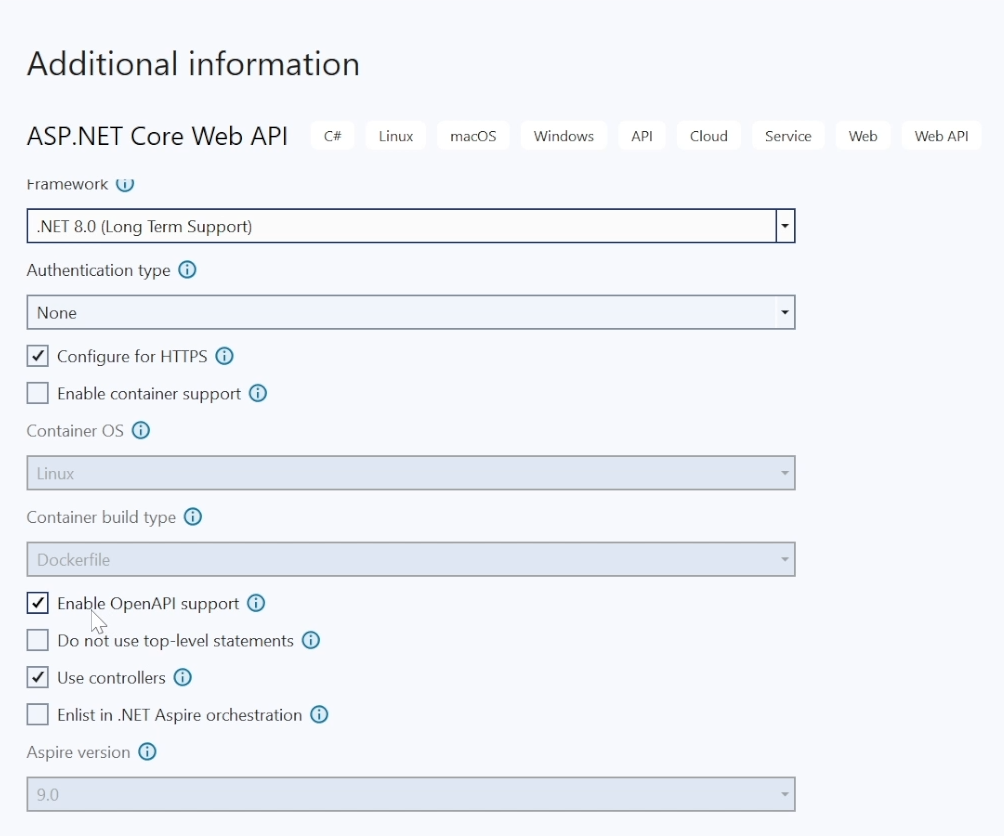
Creating a Web API in Visual Studio and enabling OpenAPI support
When you enable it, it will configure OpenAPI in the Program.cs
file. However, depending on what version you use will depend on what is configured.
Swashbuckle in .NET 8
This will configure Swashbuckle to your project. When you run the application using the Development environment, it will load the Swagger UI where you can test out the API endpoints in your application.
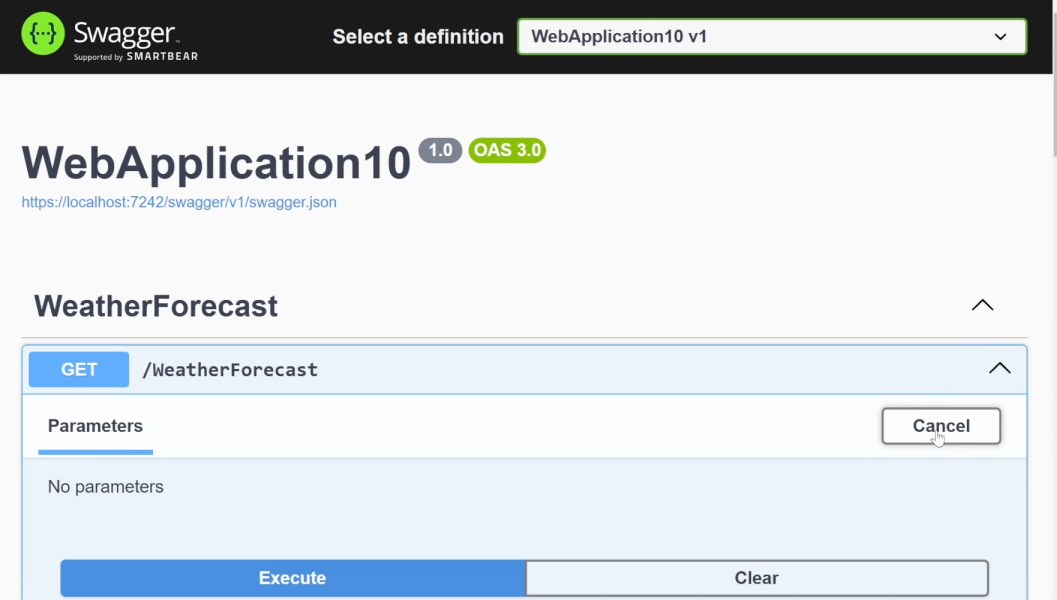
Swagger UI loaded in an ASP.NET Core Web API in .NET 8
This is configured by adding the Swashbuckle.AspNetCore
NuGet package and adding these lines of code to the Program.cs
file:
// Program.cs
var builder = WebApplication.CreateBuilder(args);
...
// Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
...
app.Run();
Things are very different in .NET 9
However when you create an ASP.NET Core Web API using .NET 9, it just adds extension methods that have a reference to OpenAPI:
// Program.cs
var builder = WebApplication.CreateBuilder(args);
...
builder.Services.AddOpenApi();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.MapOpenApi();
}
...
app.Run();
There is no reference to Swagger and the Swagger UI link gives you a 404 Not Found error response.
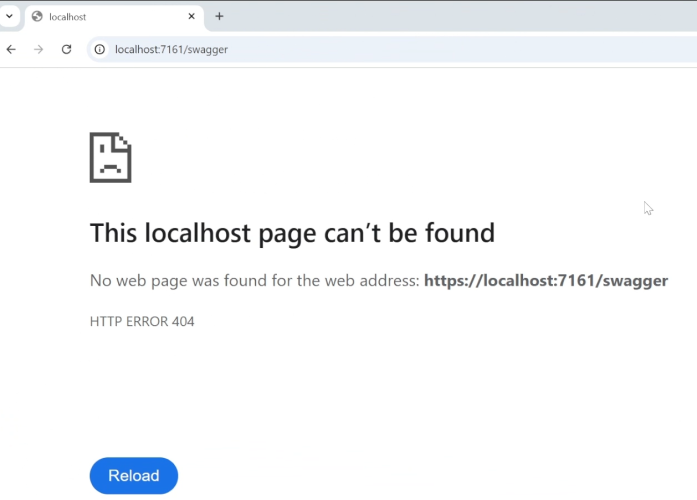
Swagger UI can't be found in .NET 9
That means that you can't test your API endpoints by default. The only thing that has been added is OpenAPI JSON documentation which you can get from /openapi/v1.json
.
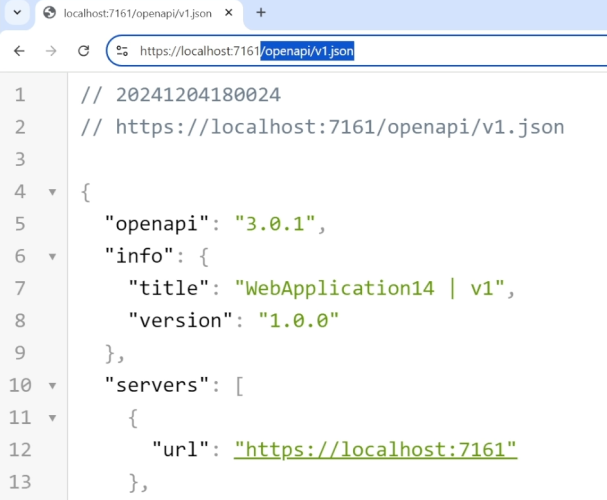
Only OpenAPI documentation is added when creating a Web API project in .NET 9
The alternatives
With Swashbuckle gone, what other ways can you test API endpoints in an application?
Postman
Postman is a good alternative as it's easy to test multiple environments. As .NET 9 Web APIs provide OpenAPI JSON documentation, we can use that link to import the endpoints in Postman.
To do that, you open the menu in the top-left hand corner and go to File and Import. Paste in the URL from your OpenAPI JSON documentation which will be https://localhost:{portnumber}/openapi/v1.json
.
When you do that, it will add a collection and save all the API endpoints added from the OpenAPI JSON document.
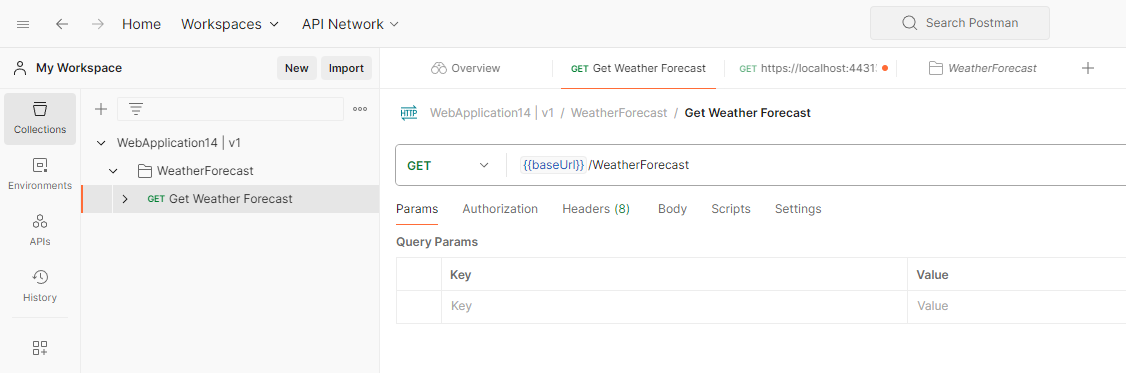
Use Postman to test your ASP.NET Core Web API endpoints
It also adds the base URL as a variable making it much easier to test in multiple environments. Just update the {{baseUrl}}
variable when testing different environments.
In addition, it stops you from accidently exposing your API endpoints in your application.
NSwag
If you want test your API endpoints inside your application, you can use NSwag. NSwag is capable of serving the Swagger UI like Swashbuckle so you'll be presented with a similar UI.
First of all, you'll need to add the NSwag.AspNetCore
NuGet package to your application. Afterwards, you'll need to call the UseSwaggerUi
extension method in Program.cs
. However, you'll need to specify the path to your OpenAPI JSON document which is openapi/v1.json
. Notice there is no forward slash at the beginning.
// Program.cs
var builder = WebApplication.CreateBuilder(args);
...
var app = builder.Build();
if (app.Environment.IsDevelopment())
{
app.MapOpenApi();
app.UseSwaggerUi(options =>
{
options.DocumentPath = "openapi/v1.json";
});
}
...
app.Run();
When you run the application and point to /swagger
, you'll be presented with the Swagger UI which works very similar to Swashbuckle.
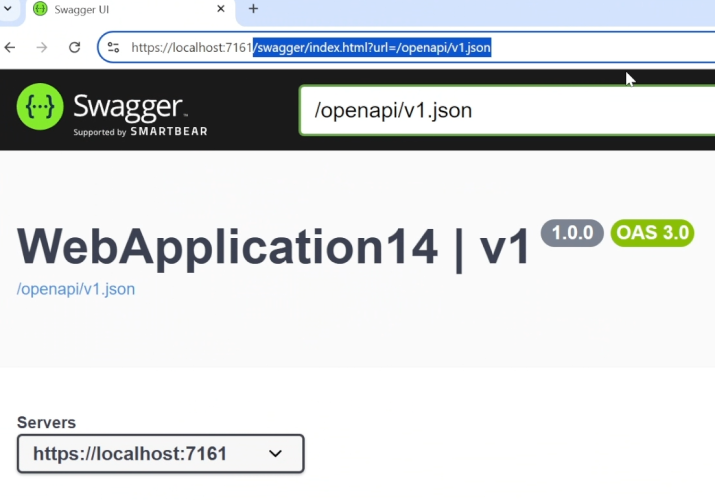
NSwag in a .NET 9 Web API using the Swagger UI
As well as that, NSwag provides NSwagStudio which allows you to import an OpenAPI JSON document and allows you to generate C# code from it. This will be useful if you are calling an external API and need to generate the code to call it.
Just add Swashbuckle back in
It's important to note that Swashbuckle still works in a .NET 9 project and you can easily configure it. Make sure you add the Swashbuckle.AspNetCore NuGet package to your project and then add the SwaggerUI configuration to your .NET 9 project in Program.cs
:
// Program.cs
var builder = WebApplication.CreateBuilder(args);
...
builder.Services.AddEndpointsApiExplorer(); // <!-- Add this line
builder.Services.AddSwaggerGen(); // <!-- Add this line
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger(); // <!-- Add this line
app.UseSwaggerUI(); // <!-- Add this line
}
...
app.Run();
When you run your application and go to /swagger
, it will show the original Swagger UI using Swashbuckle and will allow you to test your API endpoints.
Scalar: A much better API testing experience
But there is a reason why Microsoft dropped the Swagger UI and you'll probably see it if it you use Scalar. Scalar provides a much better UI design which is easier to configure, allows you to generate code to call an API endpoint in many different programming languages and allows you to add cookies, headers and query parameters to the request.
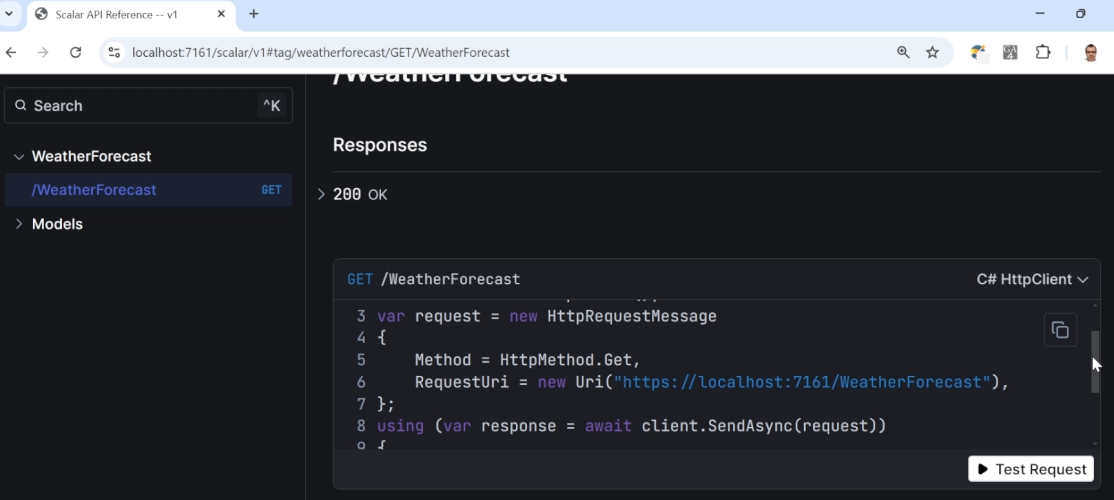
Using Scalar to test your ASP.NET Core Web API endpoints
To configure it, you add the Scalar.AspNetCore
NuGet package and then add these lines to your Program.cs
file:
// Program.cs
var builder = WebApplication.CreateBuilder(args);
...
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.MapOpenApi();
app.MapScalarApiReference(); // <-- Add this line
}
...
app.Run();
You can view the Scalar UI at /scalar/v1
when running your application.
It also allows you to configure how the Scalar UI looks and how it behaves such as changing the title name, the theme and whether the sidebar shows.
// Program.cs
using Scalar.AspNetCore;
var builder = WebApplication.CreateBuilder(args);
...
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.MapOpenApi();
app.MapScalarApiReference(options =>
{
options.WithTitle("My API");
options.WithTheme(ScalarTheme.BluePlanet);
options.WithSidebar(false);
});
}
...
app.Run();
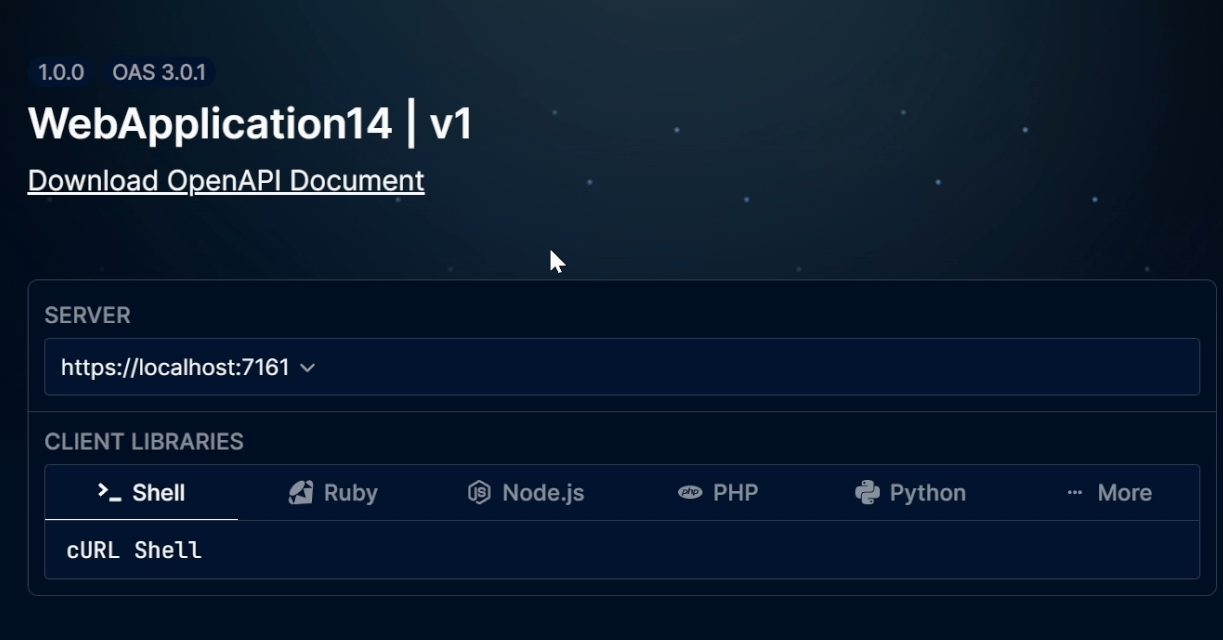
Using the Scalar UI with the BluePlanet theme
More on Scalar
Watch this video to see how Scalar works and learn about some of the other functionality that it has.
The video also covers how to set up NSwag and Swashbuckle in .NET 9 for testing your endpoints and shows you how to import an OpenAPI JSON document link into Postman.
Related pages
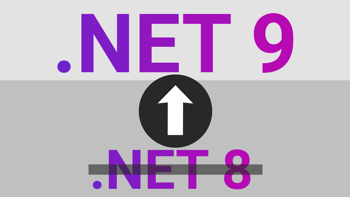
.NET 9 has been released and this is how you update
Find out how you can update your ASP.NET Core application to .NET 9 from updating Visual Studio, downloading the SDK and finding out about breaking changes.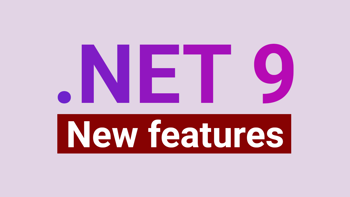